- Overview
- License
- Architecture
- System
- Performance
- Install
- Upgrade
- Uninstall
- Release Note
- Web Panel
- Config File
- Process
- Startup
- Shutdown
- Port
- Troubleshooting
- RTMP Push
- SRT Push
- SRT Pull
- UDP Push
- UDP Broadcast
- Source Stream
- Pull Stream
- Playback
- Forward
- SSL
- Snapshot
- UDP packet
- Record & NVR
- VOD
- GB28181
- WebRTC
- API
- Compare to Wowza
Location:Index Page > Document > Pull Stream
1. If you plan to pull live stream from Ti Top Streamer, Then you need to know what the url format is when pull the live stream
At persent, Ti Top Streamer support 4 type protocols: e.g. rtmp、hls、http-flv、webrtc. Below is their url format:
Protocol | url format |
rtmp | rtmp://[hostAddress]:[rtmpPort]/[applicationName]/[streamName] |
hls(ts format) | http://[hostAddress]:[httpPort]/[applicationName]/[streamName]/playlist.m3u8 |
hls(fmp4 format) | http://[hostAddress]:[httpPort]/[applicationName]/[streamName]/playlist_sfm4s.m3u8 |
hls(shift-time) | http://[hostAddress]:[httpPort]/[applicationName]/[streamName]/playlist.m3u8?shifttime=[shifttime] |
hls(NVR) | http://[hostAddress]:[httpPort]/[applicationName]/[streamName]/playlist.m3u8?start_time=[start_time]&end_time=[end_time] |
http-flv | http://[hostAddress]:[httpPort]/applicationName/[streamName]/play.flv |
[hostAddress]: It is the host address of your server running Ti Top Streamer.
[rtmpPort]: It is the port of rtmp streaming service, default is 1935.
[httpPort]: It is the port of http streaming service, default is 8080.
[applicationName]: It is the name of Application in Ti Top Streamer.
[streamName]: It is the name of a stream in the application
[shifttime]: used for shift time playback, The time difference between the start playback time and the current live streaming point, in seconds. For example,"60" means that Go back 60 seconds from the current live streaming point to start playing.
[start_time]: The start time of nvr playback, It is a unix timestamp, in milliseconds.
[end_time]: The end time of nvr playback, It is a unix timestamp, in milliseconds. Notes: The parameter "end_time" can be replaced by the parameter "duration", for example, "&duration=xxxxxxxx", It is a accurate to duration.
Suppose that the name of this Application is "live" , the streamName is "myStream" , and continue to suppose that host address is "192.168.10.102", and the port of rtmp streaming is "1935", the port of http streaming is "8080", Then the urls will be:
rtmp://192.168.10.102:1935/live/myStream http://192.168.10.102:8080/live/myStream/playlist.m3u8 http://192.168.10.102:8080/live/myStream/playlist_sfm4s.m3u8 http://192.168.10.102:8080/live/myStream/playlist.m3u8?shifttime=60 http://192.168.10.102:8080/live/myStream/playlist.m3u8?start_time=1693476761000&end_time=1693478321000 http://192.168.10.102:8080/live/myStream/play.flv
2. Ti Top Streamer support token based url anti-theft technology,through which you can achieve security protection for streaming. Here are the implementation steps:
1) Enable the feature of url anti-theft, as shown in the following figure:
Note 1:Tick the checkbox in the following image to indicate that the feature of url anti-theft is enabled (default is not enabled)
注2:Set "SharedKey", It is shared key, You can input it yourself or click the "Generate" button to automatically generate it.
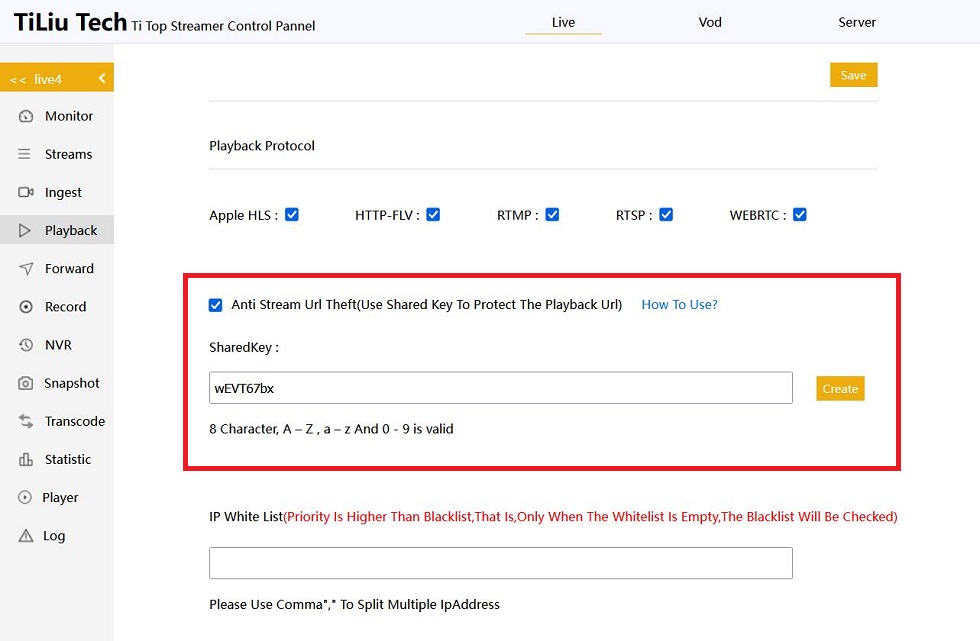
2) write code to generate streaming URLs :
In this url, Except for the host address and port, all other parts are determined based on the streaming protocol,applicationName,streamName,SharedKey,expiredTime.
The calculation process is as follows:
A. Firstly, you need to determine a URL expiration time, such as 1 minute later (URL expiration means that when you use this URL to pull a stream, the system will reject the pull request, but it does not affect connections that have already started streaming)
The URL expiration time is an absolute value, a Unix timestamp, accurate to seconds. That is the number of seconds that have passed since January 1, 1970 (midnight at UTC/GMT). Then convert it to hexadecimal format (lowercase characters)
B. Combine the sharedKey, applicationName, streamName, and expiredTime in the order described here. Be equal to(sharedKey+applicationName+streamName+expiredTime)
C. Calculate the MD5 value of the above string, Be equal to the function: md5(sharedKey+appName+streamName+expiredTime)
D. Then, you need to generate two URL query parameters, tiliuSecurityToken and tiliuExpiredTime. The former is the md5 value above, and the latter is the hexadecimal form of the URL expiration time mentioned earlier (characters in lowercase).
Notes: The rightreason reason for using prefixes like "tiliu" in these two parameters is to avoid confusion with your other custom parameters.
E. Add the above two URL query parameters to the regular streaming URL, for example:
rtmp://192.168.10.102:1935/live/myStream?tiliuSecurityToken=xxxxxxxxxxxxxxxxxxxxxxxxxxxxx&tiliuExpiredTime=xxxxxxxxxx http://192.168.10.102:8080/live/myStream/playlist.m3u8?tiliuSecurityToken=xxxxxxxxxxxxxxxxxxxxxxxxxxxxx&tiliuExpiredTime=xxxxxxxxxx http://192.168.10.102:8080/live/myStream/play.flv?tiliuSecurityToken=xxxxxxxxxxxxxxxxxxxxxxxxxxxxx&tiliuExpiredTime=xxxxxxxxxxHere is a page tool that allows you to easily generate anti-theft chain pull URLs:
Host address:
rtmp streaming port: http streaming port:
applicationName:
streamName:
sharedKey:
expiration time:(seconds)
Note: After clicking this button, a backend call will be initiated, passing in the above parameter values to obtain the corresponding streaming URL as follows:
The URL will be displayed here
The following is the backend code for this page tool, written in Node.js. You can refer to it to rewrite other language versions:
app.post('/pull/url', function (req, res) { let hostAddress = req.body.hostAddress; let rtmpPort = req.body.rtmpPort; let httpPort = req.body.httpPort; let applicationName = req.body.applicationName; let streamName = req.body.streamName; let sharedKey = req.body.sharedKey; let expiredSecond = parseInt(req.body.expiredSecond); let now = new Date(); let expiredTime = parseInt( now.getTime() / 1000 ) + expiredSecond ; let expiredTime_hex = expiredTime.toString(16); // in lowercase let originKey = sharedKey + applicationName + streamName + expiredTime_hex; let caculatedMd5 = md5(originKey) ;// md5 function let rtmp_base_url = "rtmp://"+hostAddress+":"+rtmpPort+"/"+applicationName+"/"+streamName ; let rtmp_url = rtmp_base_url+"?tiliuSecurityToken="+caculatedMd5+"&tiliuExpiredTime="+expiredTime_hex; let hls_base_url = "http://"+hostAddress+":"+httpPort+"/"+applicationName+"/"+streamName+"/playlist.m3u8" ; let hls_url = hls_base_url+"?tiliuSecurityToken="+caculatedMd5+"&tiliuExpiredTime="+expiredTime_hex; let flv_base_url = "http://"+hostAddress+":"+httpPort+"/"+applicationName+"/"+streamName+"/play.flv" ; let flv_url = flv_base_url+"?tiliuSecurityToken="+caculatedMd5+"&tiliuExpiredTime="+expiredTime_hex; res.status(200).json({"code":200,"rtmpUrl":rtmp_url,"hlsUrl":hls_url,"flvUrl":flv_url}); });We strongly recommend that you place the code logic for generating pull URLs on the server side, as in our example above, by obtaining them from the backend through an api.