- Overview
- License
- Architecture
- System
- Performance
- Install
- Upgrade
- Uninstall
- Release Note
- Web Panel
- Config File
- Process
- Startup
- Shutdown
- Port
- Troubleshooting
- RTMP Push
- SRT Push
- SRT Pull
- UDP Push
- UDP Broadcast
- Source Stream
- Pull Stream
- Playback
- Forward
- SSL
- Snapshot
- UDP packet
- Record & NVR
- VOD
- GB28181
- WebRTC
- API
- Compare to Wowza
Location:Index Page > Document > RTMP Push
1. If you use rtmp to push streams to Ti Top Streamer, then you need to know what the rtmp target URL is
Notes:Firstly, you need an live application with a source type of rtmp push, about the source type, Please read overview
url format of rtmp target :
rtmp://[hostAddress]:[rtmpPort]/[applicationName]/[streamName][hostAddress]: It is the host address of your server which Ti Top Streamer is running.
[rtmpPort]: It is the rtmp streaming port of your server which Ti Top Streamer is running,default is 1935.
[applicationName]: it is the name of application in Ti Top Streamer.
[streamName]: It is the name of your live stream , also be called as "key" in obs studio.
Suppose that the name of this application is "live", and streamName is "myStream", and continue suppose that hostAddress is "192.168.10.102" and port is "1935", then rtmp target url will be :
rtmp://192.168.10.102:1935/live/myStream
2. Ti Top Streamer supports token based url anti-theft technology, through which you can achieve security protection for streaming. Here are the implementation steps:
1) Enable the anti-theft function, as shown in the following picture:
Note 1:Tick the checkbox in the following image to indicate that the url anti-theft function is enabled (default is not enabled)
Note 2:Set a "SharedKey", It is a shared key, You can input it yourself or click the "Generate" button to automatically generate it.
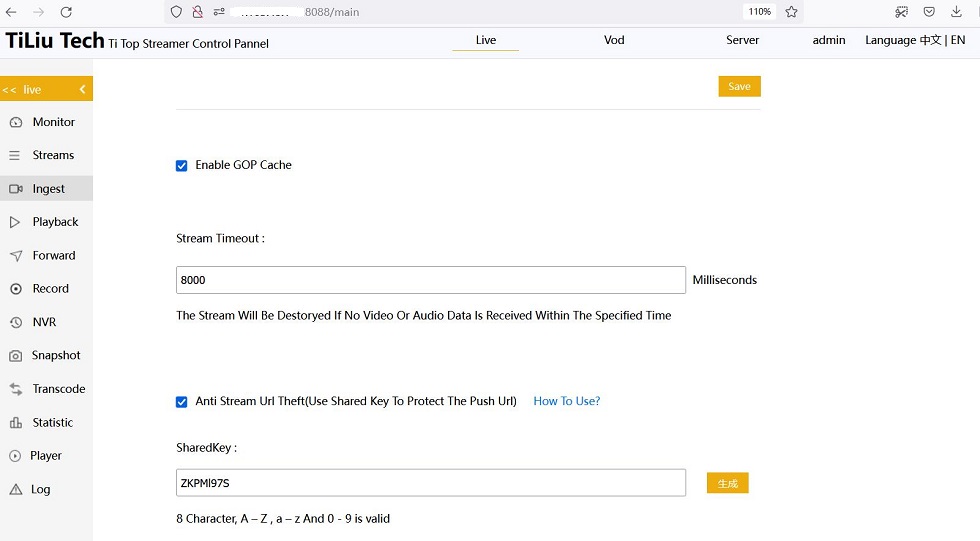
2) write code to generate RTMP streaming URL
In the URL of RTMP streaming, apart from the host address and port, other parts are determined based on applicationName, streamName, SharedKey, and expiredTime (expiration time).
The calculation process is as follows:
A. Firstly, you need to determine a URL expiration time, such as 1 minute later (URL expiration means that when you use this URL to push a stream, the system will reject the push request, but it does not affect the connections that have already started pushing)
The URL expiration time is an absolute value, a Unix timestamp, accurate to seconds. That is the number of seconds that have passed since January 1, 1970 (midnight UTC/GMT). Then convert it to hexadecimal format (lowercase characters).
B. Linking sharedKey, applicationName, streamName, and expiredTime in the order described here is equivalent to (sharedKey+applicationName+streamName+expiredTime)
C. Calculate the MD5 value of the above string, which is equivalent to MD5(sharedKey+appName+streamName+expiredTime)
D. Then you need to generate two URL query parameters: "tiliuSecurityToken" and "tiliuExpiredTime", The former is the MD5 value mentioned above, while the latter is the hexadecimal form of the URL expiration time mentioned earlier(use lowercase characters).
Notes: The reason for using prefixes like "tiliu" in these two parameters is to avoid confusion with your other custom parameters.
E. Add the above two URL query parameters to the regular RTMP streaming URL, for example:
rtmp://192.168.10.102:1935/live/myStream?tiliuSecurityToken=xxxxxxxxxxxxxxxxxxxxxxxxxxxxx&tiliuExpiredTime=xxxxxxxxxxHere is a web page tool that allows you to easily generate anti-theft push url:
Host Address:
Port:
applicationName:
streamName:
sharedKey:
Expired Second:(Second,and we will convert it to absolute time)
Note: After clicking this button, a backend call will be initiated, passing in the above parameter values to obtain a streaming URL as follows:
rtmp target url will be displayed here
If you want to actually test streaming, there are many tools to choose from, such as FFMpeg, OBS Studio, and Vmix. The following example is using FFMpeg to push audio and video content from an mp4 file (sample. mp4)
ffmpeg -re -i sample.mp4 -vcodec copy -acodec copy -f flv "the rtmp url at above"
You can get FFMpeg from here : http://ffmpeg.org/download.html
The following is the backend code for the page tool that generated the RTMP URL in the previous step, written in Node.js. You can refer to it to rewrite other language versions:
app.post('/rtmp/push/url', function (req, res) { let hostAddress = req.body.hostAddress; let rtmpPort = req.body.rtmpPort; let applicationName = req.body.applicationName; let streamName = req.body.streamName; let sharedKey = req.body.sharedKey; let expiredSecond = parseInt(req.body.expiredSecond); let now = new Date(); let expiredTime = parseInt( now.getTime() / 1000 ) + expiredSecond ; let expiredTime_hex = expiredTime.toString(16); // lowcase let originKey = sharedKey + applicationName + streamName + expiredTime_hex; let caculatedMd5 = md5(originKey) ; //md5 library let base_url = "rtmp://"+hostAddress+":"+rtmpPort+"/"+applicationName+"/"+streamName ; let url = base_url+"?tiliuSecurityToken="+caculatedMd5+"&tiliuExpiredTime="+expiredTime_hex; res.status(200).json({"code":200,"url":url}); });We strongly recommend that you place the code logic for generating the streaming URL on the server side, as in our example above, by obtaining it from the backend through an interface.